Read Only Letters in a String C
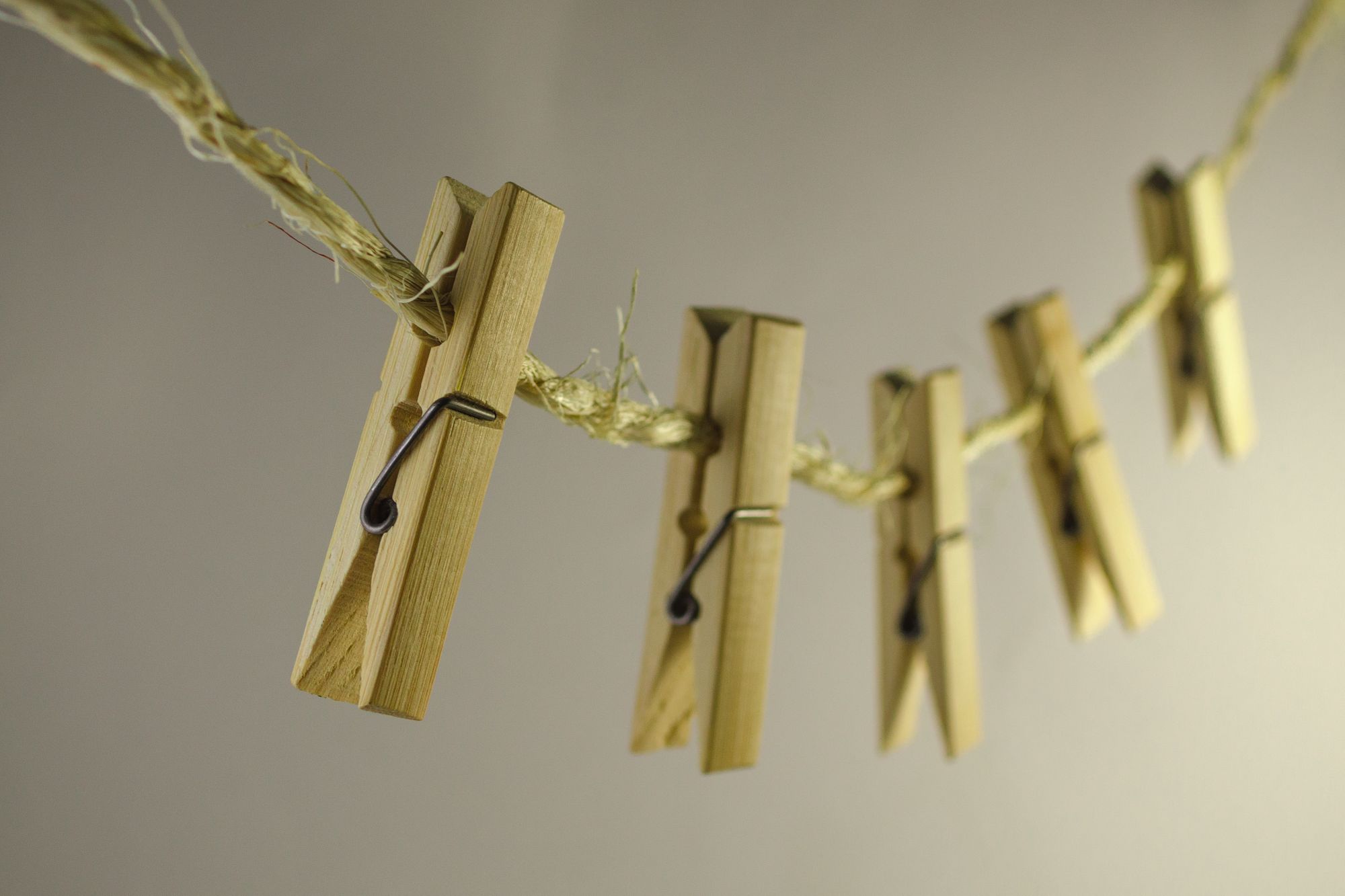
Computers store and process all kinds of data.
Strings are just ane of the many forms in which information is presented and gets processed by computers.
Strings in the C programming language work differently than in other modern programming languages.
In this commodity, y'all'll larn how to declare strings in C.
Before doing so, you'll go through a bones overview of what data types, variables, and arrays are in C. This style, you'll understand how these are all connected to one another when it comes to working with strings in C.
Knowing the basics of those concepts will so help you improve sympathise how to declare and work with strings in C.
Permit'south get started!
Data types in C
C has a few born information types.
They are int
, brusque
, long
, bladder
, double
, long double
and char
.
As yous see, there is no built-in string or str (brusque for string) information blazon.
The char
data blazon in C
From those types yous just saw, the only way to utilise and present characters in C is by using the char
information type.
Using char
, you are able to to represent a single character – out of the 256 that your computer recognises. Information technology is most commonly used to represent the characters from the ASCII chart.
The unmarried characters are surrounded past single quotation marks.
The examples below are all char
south – fifty-fifty a number surrounded by unmarried quoation marks and a unmarried space is a char
in C:
'D', '!', 'v', 'l', ' '
Every single letter, symbol, number and space surrounded by single quotation marks is a single piece of character data in C.
What if you want to present more than one single graphic symbol?
The post-obit is not a valid char
– despite existence surrounded past single quotation marks. This is considering it doesn't include only a single character inside the single quotation marks:
'freeCodeCamp is awesome'
When many single characters are strung together in a group, like the sentence you see in a higher place, a cord is created. In that case, when you are using strings, instead of single quotation marks you should only utilise double quotation marks.
"freeCodeCamp is awesome"
How to declare variables in C
So far you've seen how text is presented in C.
What happens, though, if you desire to store text somewhere? After all, computers are really good at saving information to retention for later retrieval and utilise.
The way you shop data in C, and in most programming languages, is in variables.
Substantially, you can think of variables as boxes that concord a value which tin can change throughout the life of a program. Variables classify infinite in the figurer'due south memory and allow C know that you lot want some space reserved.
C is a statically typed linguistic communication, meaning that when you create a variable you have to specify what data type that variable will be.
There are many unlike variable types in C, since at that place are many unlike kinds of data.
Every variable has an associated data blazon.
When yous create a variable, you first mention the blazon of the variable (wether it will hold integer, float, char or any other data values), its proper noun, and then optionally, assign information technology a value:
#include <stdio.h> int main(void){ char letter = 'D'; //creates a variable named letter of the alphabet //it holds only values of type char // the single grapheme 'D' is assigned to letter }
Be careful not to mix information types when working with variables in C, as that will cause errors.
For intance, if yous try to alter the example from above to use double quotation marks (call back that chars just use single quotation marks), you'll get an error when you compile the code:
#include <stdio.h> int primary(void){ char letter = "D"; //output: test.c:four:6: warning: incompatible pointer to integer conversion initializing 'char' with an expression of type 'char [2]' [-Wint-conversion] char letter = "D"; ^ ~~~ 1 alert generated. }
As mentioned earlier on, C doesn't take a built-in string data blazon. That also means that C doesn't have string variables!
How to create arrays in C
An array is essentially a variable that stores multiple values. It'south a drove of many items of the aforementioned type.
As with regular variables, there are many different types of arrays because arrays can hold simply items of the same data blazon. In that location are arrays that concord just int
s, just bladder
s, and then on.
This is how yous define an array of ints
south for example:
int numbers[iii];
Beginning you lot specify the information blazon of the items the array will hold. Then you give information technology a name and immediately later the name yous also include a pair of foursquare brackets with an integer. The integer number speficies the length of the assortment.
In the example above, the array can hold 3
values.
Later on defining the array, y'all tin assign values individually, with square bracket notation, using indexing. Indexing in C (and most programming languages) starts at 0
.
//Define the array; information technology tin can concord 3 values int numbers[3]; //assign the 1st item of the numbers assortment the value of 1 int numbers[0] = 1; //assign the second detail of the numbers assortment the value of ii int numbers[1] = 2; //assing the 3rd item of the numbers assortment the value of iii int numbers[ii] = 3;
You reference and fetch an item from an array by using the name of the array and the item'south index in foursquare brackets, like so:
numbers[ii]; // returns the value 3
What are character arrays in C?
So, how does everything mentioned so far fit together, and what does information technology have to practice with initializing strings in C and saving them to retentivity?
Well, strings in C are actually a blazon of array – specifically, they are a character assortment
. Strings are a collection of char
values.
How strings work in C
In C, all strings end in a 0
. That 0
lets C know where a string ends.
That string-terminating nil is called a string terminator. You may also come across the term goose egg zero used for this, which has the same meaning.
Don't misfile this final nix with the numeric integer 0
or even the character '0'
- they are not the aforementioned thing.
The string terminator is added automatically at the finish of each string in C. But it is non visible to united states – information technology'south only ever at that place.
The string terminator is represented like this: '\0'
. What sets it apart from the graphic symbol '0'
is the backslash it has.
When working with strings in C, information technology's helpful to pic them ever ending in nothing zero and having that extra byte at the finish.
Each character takes upward one byte in retention.
The string "howdy"
, in the picture in a higher place, takes up half-dozen bytes
.
"Hello" has five letters, each ane taking upward 1 byte of space, and then the cypher cypher takes upward one byte too.
The length of strings in C
The length of a string in C is only the number of characters in a word, without including the cord terminator (despite information technology always being used to terminate strings).
The string terminator is non accounted for when yous want to find the length of a cord.
For example, the string freeCodeCamp
has a length of 12
characters.
Merely when counting the length of a string, you must e'er count any blank spaces too.
For example, the string I code
has a length of vi
characters. I
is 1 character, code
has four characters, and then there is 1 bare space.
So the length of a string is non the same number every bit the number of bytes that it has and the corporeality of memory space it takes up.
How to create character arrays and initialize strings in C
The first step is to utilise the char
data type. This lets C know that you want to create an array that will hold characters.
Then you give the array a name, and immediatelly after that y'all include a pair of opening and closing square brackets.
Inside the square brackets you'll include an integer. This integer will be the largest number of characters you desire your string to be including the string terminator.
char city[7];
You can initialise a string one character at a time like so:
#include <stdio.h> int main(void) { char city[7]; urban center[0] = 'A'; city[1] = 't'; city[ii] = 'h'; city[3] = 'e'; city[iv] = 'north'; city[5] = 's'; metropolis[6] = '\0'; //don't forget this! printf("I alive in %s",urban center); }
But this is quite time-consuming. Instead, when y'all first define the grapheme array, you lot have the option to assign it a value directly using a string literal in double quotes:
#include <stdio.h> int main(void){ char city[7] = "Athens"; //defines a character array named urban center //it can concur a cord up to seven characters INCLUDING the string terminator //the value "Athens" is assigned when the grapheme array is being defined //this is how you print the grapheme array value printf("I alive in %southward",city); }
If you want, istead of including the number in the square brackets, you tin can simply assign the grapheme array a value.
Information technology works exactly the same equally the instance above. It will count the number of characters in the value y'all provide and automatically add together the naught cypher grapheme at the end:
char urban center[] = "Athens"; //"Athens" has a length of 6 characters //"Athens" takes upward vii bytes in memory,with the cypher cypher included /* char city[7] = "Athens"; is equal to char metropolis[] = "Athens"; */
Remember, you always need to reserve enough infinite for the longest string you lot want to include plus the string terminator.
If you want more room, demand more than memory, and plan on changing the value after, include a larger number in the foursquare brackets:
char city[15] = "Athens"; /* The city graphic symbol array volition now exist able to agree fifteen characters (including the null zero) In this case, the remaining eight (15 - vii) places will exist empty Yous'll be able to reassign a value up to 15 characters (including null nil every bit always) */
How to change the contents of a character array
So, yous know how to initialize strings in C. What if you want to change that string though?
You cannot simply employ the assignment operator (=
) and assign it a new value. You can only do that when you kickoff define the graphic symbol array.
As seen before on, the way to access an item from an array is by referencing the assortment's name and the item'due south index number.
So to modify a string, you lot can modify each grapheme individually, one past one:
#include <stdio.h> int chief(void) { char city[7] = "Athens"; printf("I live in %due south",city); //irresolute each character individually means you have to apply single quotation marks //the new value has to accept upward 7 bytes of memory //indexing starts at 0, the first character has an index of 0 urban center[0] = 'L'; metropolis[ane] = 'o'; city[ii] = 'due north'; metropolis[3] = 'd'; city[4] = 'o'; urban center[v] = 'northward'; urban center[6] = '\0'; //DON'T FORGET THIS! printf("\nBut now I live in %s",city); } //output: //I live in Athens //But at present I alive in London
That method is quite cumbersome, time-consuming, and mistake-prone, though. Information technology definitely is not the preferred way.
Yous tin instead utilize the strcpy()
part, which stands for string copy
.
To utilise this part, you have to include the #include <string.h>
line after the #include <stdio.h>
line at the top of your file.
The <string.h>
file offers the strcpy()
office.
When using strcpy()
, you first include the proper name of the grapheme array and and so the new value y'all want to assign. The strcpy()
part automatically add the string terminator on the new string that is created:
#include <stdio.h> #include <string.h> int chief(void) { char city[15] = "Athens"; strcpy(metropolis,"Barcelona"); printf("I am going on vacation to %s",city); //output: //I am going on holiday to Barcelona }
Conclusion
And there you accept information technology. At present you know how to declare strings in C.
To summarize:
- C does non have a built-in string office.
- To work with strings, you take to employ grapheme arrays.
- When creating character arrays, leave enough space for the longest cord you'll desire to shop plus account for the cord terminator that is included at the end of each string in C.
- To place or change strings in character arrays you either:
- Define the assortment and then assign each individual character element one at a fourth dimension.
- OR ascertain the array and initialize a value at the same time.
- When changing the value of the cord, you lot can use the
strcpy()
function later on yous've included the<cord.h>
header file.
If you lot want to learn more about C, I've written a guide for beginners taking their starting time steps in the language.
It is based on the offset couple of weeks of CS50'southward Introduction to Reckoner Science course and I explain some fundamental concepts and go over how the language works at a loftier level.
You can also lookout man the C Programming Tutorial for Beginners on freeCodeCamp's YouTube aqueduct.
Thanks for reading and happy learning :)
Learn to code for complimentary. freeCodeCamp's open source curriculum has helped more than than 40,000 people get jobs equally developers. Become started
Source: https://www.freecodecamp.org/news/c-string-how-to-declare-strings-in-the-c-programming-language/
0 Response to "Read Only Letters in a String C"
Post a Comment